Quizzler (2)
해당 강의 내용을 정리하기전. 강의에서 Structure가 필요하기에 그부분을 정리하고자한다.
구조체 Structure
- 정의
1
struct Mystruct { }
구조체를 만들때는 처음부터 이름을 대문자로 표기한다
- 우리가 평상시 만들던 CamelCase가 아니다!
- 일종의 Data Type으로 생각을 하면 되겠다. - ex) Int, Float, Double ….
Structure를 한번 만들어 보았다.
1
2
3
4
5
6
7
8
9
10
struct Town {
let name = "HaroldLand"
var citizens = ["Harold", "Angela"]
var resources = ["Grain" : 100, "Ore" : 42, "Wool" : 75]
}
var myTown = Town()
var myTown = Town()을 통해 이제 myTown이라는 매개변수를 이용해 structure에 접근을 할 수 있게되었다.
또한 추가를 하고싶을때는 append를 사용한다
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
struct Town {
let name = "HaroldLand"
var citizens = ["Harold", "Angela"]
var resources = ["Grain" : 100, "Ore" : 42, "Wool" : 75]
}
var myTown = Town()
myTown.citizens.append("Keanu Reeves")
print(myTown.citizensa)
print(myTown.citizens.count)
물론 structure안에 함수도 넣을 수 있다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
struct Town {
let name = "HaroldLand"
var citizens = ["Harold", "Angela"]
var resources = ["Grain" : 100, "Ore" : 42, "Wool" : 75]
func fortify() {
print("Defences increased!")
}
}
var myTown = Town()
myTown.citizens.append("Keanu Reeves")
print(myTown.citizens)
print(myTown.citizens.count)
myTown.fortify() //호출도 가능하다.
initialize -> property를 Ininitailization 해준다
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
struct Town {
let name : String
var citizens : [String]
var resources : [String : Int]
init (townName : String, people : [String], stats : [String : Int])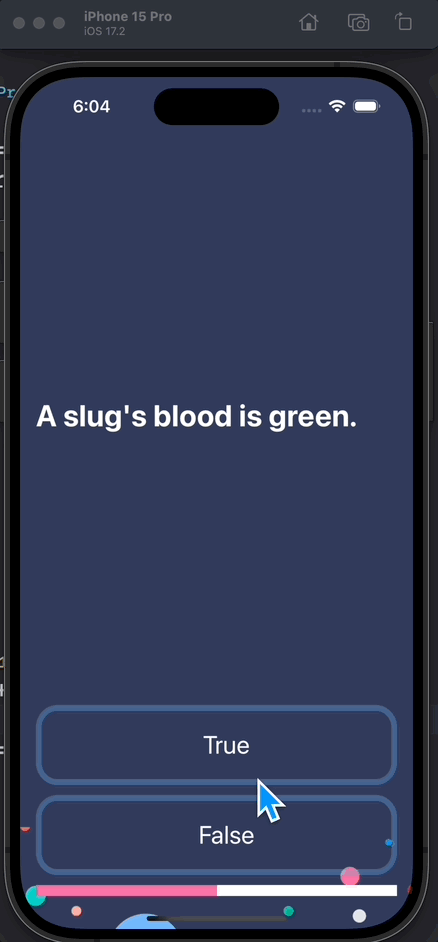
{
name = townName
citizens = people
resources = stats
}
func fortify() {
print("Defences increased!")
}
}
매개변수를 같게 해보자
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
struct Town {
let name : String
var citizens : [String]
var resources : [String : Int]
init (name : String, citizens : [String], resources : [String : Int]){
self.name = name
self.citizens = citizens
self.resources = resources
}
func fortify() {
print("Defences increased!")
}
}
self를 붙이지않고 name = name이런식으로 하게되면 에러가 발생한다. structure의 name이 상수이기때문.
self를 붙이면서 해소가 되는데 self는 구조체를 가리키는걸로 생각하면 쉽다.
다시 강의정리로 돌아와서 현재 2차원 배열로 되어있는 quiz를 파일을 새로 만들어서 거기에 별도로 넣어보자.
우클릭 -> New File -> Swift File
그리고 init을 적고 Initializing을 해주었다.
그리고나서 문제와 답을 가지고 있는 배열을 structure에 맞게 바꿔주었다.
그리고 우리가 문제를 맞췄는지 틀렸는지에 관해 console에서만 확인 할 수있던것을 버튼에 색을 주어 나타내었다.
버튼 색만 변경을 해주어서 다시 원래대로 돌아오지 않았다.
1
2
3
4
5
func updateUI() {
questionLabel.text = quiz[questionNumber].text
trueButton.backgroundColor = UIColor.clear
falseButton.backgroundColor = UIColor.clear
}
그래서 버튼의 uicolor를 clear해주었다. 과연?
이제는 아예 색이 보이지 않는다.
다시한번 해보자.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var questionLabel: UILabel!
@IBOutlet weak var progresBar: UIProgressView!
@IBOutlet weak var trueButton: UIButton!
@IBOutlet weak var falseButton: UIButton!
let quiz = [
Question(q: "A slug's blood is green.", a: "True"),
Question(q: "Approximately one quarter of human bones are in the feet.", a: "True"),
Question(q: "The total surface area of two human lungs is approximately 70 square metres.", a: "True"),
Question(q: "In West Virginia, USA, if you accidentally hit an animal with your car, you are free to take it home to eat.", a: "True"),
Question(q: "In London, UK, if you happen to die in the House of Parliament, you are technically entitled to a state funeral, because the building is considered too sacred a place.", a: "False"),
Question(q: "It is illegal to pee in the Ocean in Portugal.", a: "True"),
Question(q: "You can lead a cow down stairs but not up stairs.", a: "False"),
Question(q: "Google was originally called 'Backrub'.", a: "True"),
Question(q: "Buzz Aldrin's mother's maiden name was 'Moon'.", a: "True"),
Question(q: "The loudest sound produced by any animal is 188 decibels. That animal is the African Elephant.", a: "False"),
Question(q: "No piece of square dry paper can be folded in half more than 7 times.", a: "False"),
Question(q: "Chocolate affects a dog's heart and nervous system; a few ounces are enough to kill a small dog.", a: "True")
// Question(q: "Four + Two is equal to Six.", a: "True"),
// Question(q: "Five - Three is greater than One.", a: "True"),
// Question(q: "Three + Eight is less than Ten.", a: "False")
]
var questionNumber = 0
var timer = Timer()
override func viewDidLoad() {
super.viewDidLoad()
updateUI()
}
@IBAction func answerButtonPressed(_ sender: UIButton) {
let userAnswer = sender.currentTitle // true or false
let actualAnswer = quiz[questionNumber].answer
//let actualQuestion = quiz[questionNumber]
//let actualAnswer = actualQuestion.answer
if userAnswer == actualAnswer {
sender.backgroundColor = UIColor.green
} else {
sender.backgroundColor = UIColor.red
}
// if questionNumber == 2 {
// questionNumber = 0
// }
//
// questionNumber += 1
if questionNumber + 1 < quiz.count {
questionNumber += 1
} else {
questionNumber = 0
}
timer = Timer.scheduledTimer(timeInterval: 1.0, target: self, selector: #selector(updateUI), userInfo: nil, repeats: true)
//updateUI()
}
@objc func updateUI() {
questionLabel.text = quiz[questionNumber].text
trueButton.backgroundColor = UIColor.clear
falseButton.backgroundColor = UIColor.clear
}
}
Timer를 써야할거같았는데 혹시 다른방법이 있나 했는데 결국 없었다.
timer를 만들어서 selector에 updateUI를 해주었다. 이때 selecter에 updateUI가 들어가면서 func updateUI~ 이랬떤 부분을
@objc func updateUI로 바꾸어 주었다. selector가 objective-C에 의존하기 때문.
시간의 편차가 생기긴 하지만 그래도 작동은 된다.
알고보니 repeats 때문인듯하다. 0.2로 바꾸어주고 repeats도 false를 해주니 잘된다.
아마 시간편차는 타이머가 반복으로 되면서 꼬였던것으로 보인다.
progress bar를 추가하여 문제에따른 진행률을 해보자.